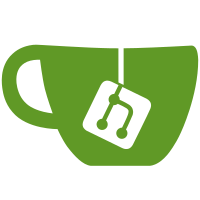
Ignored only the rules about continuing / hanging indentation. Also, added __init__.py to tests so that pylint discovers them. (I don't buy pytest's BS about installing your package.)
27 lines
701 B
Python
27 lines
701 B
Python
import os
|
|
import yaml
|
|
|
|
|
|
def merge(left, right):
|
|
for key in right:
|
|
if key in left:
|
|
if isinstance(left[key], dict) and isinstance(right[key], dict):
|
|
merge(left[key], right[key])
|
|
elif left[key] != right[key]:
|
|
left[key] = right[key]
|
|
else:
|
|
left[key] = right[key]
|
|
return left
|
|
|
|
|
|
def read_config():
|
|
with open('../config.yaml.dist') as handle:
|
|
ret = yaml.load(handle.read())
|
|
if os.path.exists('../config.yaml'):
|
|
with open('../config.yaml') as handle:
|
|
ret = merge(ret, yaml.load(handle.read()))
|
|
return ret
|
|
|
|
|
|
config = read_config() # pylint: disable=invalid-name
|